Automating Confidence
By @johnkpaul
Who?
John K. Paul
Penton Media
I ♥ JavaScript
@johnkpaul
john@johnkpaul.com
johnkpaul.com
Validation
1. // Backbone example
2. App.BaseView = Backbone.View.extend({
3. render: function(){
4.
5. }
6. );
7.
8. // node example
9. fs.readFile('foo', function(err, file){
10. handleSuccess(file.toUpperCase)());
11. });
1. App.BaseView = Backbone.View.extend({
2. render: function(){
3.
4. }
5. );
6.
7. require('fs').readFile('foo', function(err, file){
8. handleSuccess(file.toUpperCase)());
9. });
>> Line 5: Unexpected token )
>> Line 8: Unexpected token )
- esprima
- grunt-jsvalidate
- Google Closure
YOUR IDE CAN DO THAT
Linting
- common semantic mistakes
- JSHint/ESLint/JSLint
- code style
- JSCS
Linting
1 (function(){
2
3 if (value = 1) {
4 doSomething(value)
5 }
6
7 }());
line 3, col 3, Missing "use strict" statement.
line 3, col 16, Expected a conditional expression
and instead saw an assignment.
line 4, col 23, Missing semicolon.
Linting Examples
1. (function(){
2.
3. test();
4.
5. var test = function(){};
6. }());
[L2:C3] Missing "use strict" statement.
test();
[L4:C12] 'test' was used before it was defined.
var test = function(){};
Decisions
Complexity
Path counting
1. (function () {
2. if (test1()) {
3. doSomething();
4. } else if (test2()) {
5. doSomethingElse();
6. } else if (test3()) {
7. doSomethingElse2();
8. }
9. }());
line 1, col 11, This function's cyclomatic
complexity is too high. (4)
Beautification
1. if ( test1())
2. {
3. doSomething();
4. } else if (test2()) {
5. doSomethingElse();
6. }
7. else if (test4())
8. {
9. doSomethingElse2();
10. }
1. if (test1()) {
2. doSomething();
3. } else if (test2()) {
4. doSomethingElse();
5. } else if (test4()) {
6. doSomethingElse2();
7. }
Beautification
- EditorConfig
- codepainter
- js-beautify
- esformatter
Enforcement
pre commit hooks
#!/bin/sh
# run script to check if commit is valid
node ./good-to-commit.js
RETVAL=$?
if [ $RETVAL -ne 0 ]
then
exit 1
fi
TypeScript
1. function add(a: number, b: number) {
2. return a + b;
3. }
4.
5. add("a", "b");
Supplied parameters do not match any signature of call target.
Could not select overload for 'call' expression.
(a: number, b: number): number
TypeScript compiler
- One tool for syntax validation and most of linting
- Compile time structural type validation
- Strict superset of JavaScript
- Significantly inspired by ES6
- INTELLISENSE
What you should do
- Add validation and linting
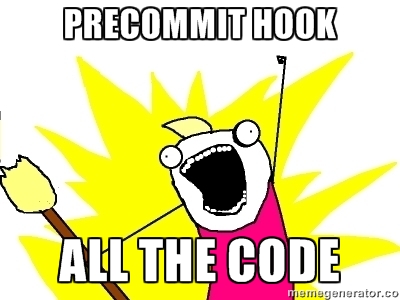
Thank you!
By @johnkpaul
john@johnkpaul.com